Spring Boot Security
Introduction
Security is a crucial aspect of any web application, and it is especially important for applications that handle sensitive data. Spring Boot provides a comprehensive security framework that makes it easy to secure your applications against a wide range of threats. In this blog post, we will explore how Spring Boot handles security.
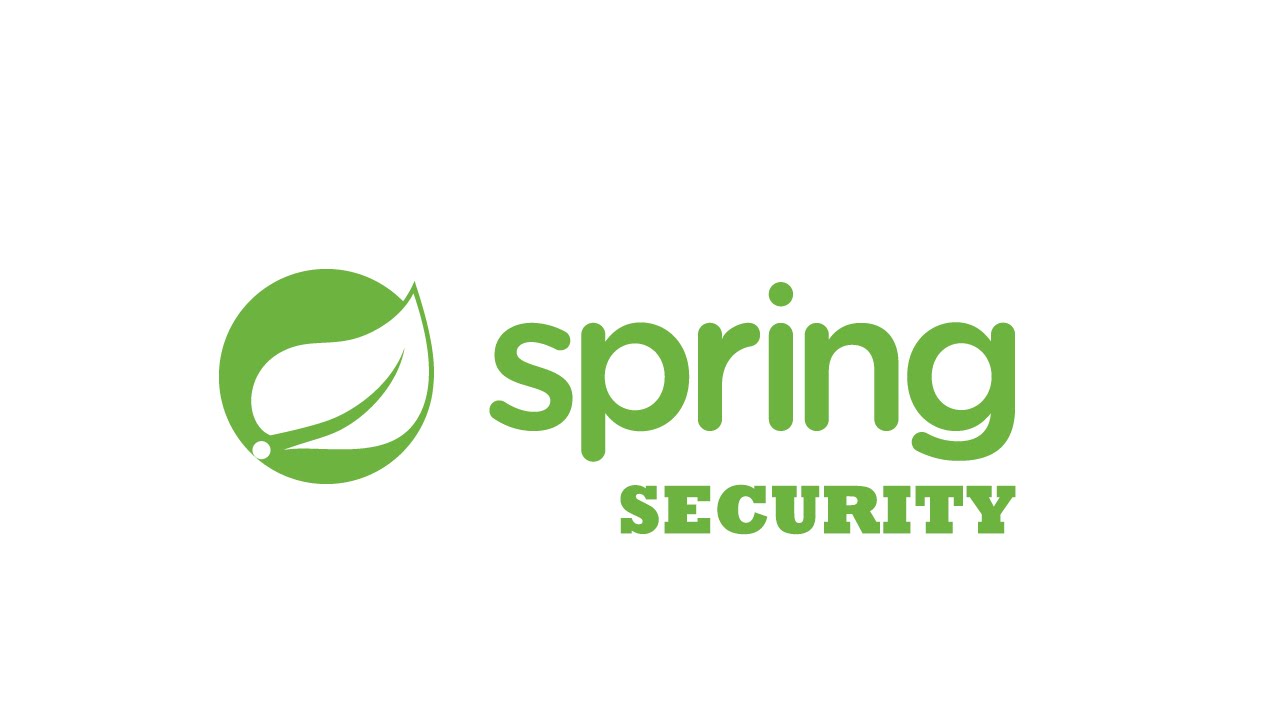
Setting Up Spring Boot Security
Before we dive into the details of how Spring Boot handles security, let’s take a look at how to set it up. Spring Boot provides a starter dependency that includes everything you need to get started with security. To add security to your application, simply add the following dependency to your pom.xml file:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
Once you have added the dependency, Spring Boot will automatically configure a basic security setup that requires users to authenticate before accessing any part of the application.
Configuring Spring Boot Security
By default, Spring Boot uses HTTP Basic authentication to secure your application. However, you can configure Spring Boot to use a variety of authentication methods, including form-based authentication, LDAP, OAuth, and more.
To configure Spring Boot security, you can create a class that extends the WebSecurityConfigurerAdapter class. This class provides a number of methods that you can use to configure various aspects of security, such as authentication, authorization, and more.
Here’s an example of how to configure Spring Boot security using the WebSecurityConfigurerAdapter class:
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/public/**").permitAll()
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.permitAll()
.and()
.logout()
.logoutSuccessUrl("/login?logout")
.permitAll();
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth
.inMemoryAuthentication()
.withUser("user").password("{noop}password").roles("USER")
.and()
.withUser("admin").password("{noop}password").roles("USER", "ADMIN");
}
}
In this example, we’ve created a SecurityConfig class that extends WebSecurityConfigurerAdapter. We've overridden the configure(HttpSecurity http) method to configure the security settings for HTTP requests. We've specified that any request that matches the /public/** pattern should be permitted without authentication, while any other request should require authentication. We've also specified that we want to use form-based authentication, and we've provided a custom login page. Finally, we've specified a custom logout URL.
In the configure(AuthenticationManagerBuilder auth) method, we've specified a list of users and their roles. In this example, we've created two users: user and admin. Both users have the password password, and the admin user has an additional ADMIN role.
Using Spring Boot Security in Your Application
Once you have configured Spring Boot security, you can use it to secure your application by adding security annotations to your controllers and methods. Spring Boot provides a number of annotations that you can use to secure your application, including @Secured, @RolesAllowed, and @PreAuthorize.
Here’s an example of how to use the @PreAuthorize annotation to secure a method:
@RestController
@RequestMapping("/api")
public class MyController {
@GetMapping("/users")
@PreAuthorize("hasRole('ADMIN')")
public List<User> getUsers() {
// code to get a list of users
}
}
In this example, we’ve added the @PreAuthorize("hasRole('ADMIN')") annotation to the getUsers() method. This annotation specifies that only users with the ADMIN role are allowed to access this method.
Conclusion
Spring Boot provides a powerful and flexible security framework that makes it easy to secure your applications against a wide range of threats. With Spring Boot security, you can easily configure authentication and authorization, use a variety of authentication methods, and secure your controllers and methods with a variety of annotations. By following the guidelines outlined in this blog post, you can use Spring Boot security to ensure that your applications are secure and protected against unauthorized access.
Science and TechnologyYou may be interested in these jobs
-
Line Cook>
Found in: Lensa US 4 C2 - 5 hours ago
Wahlburgers Minneapolis, United StatesWahlburgers - Mall of America [Kitchen Staff / Grill Cook / Prep Cook] As a Line Cook at Wahlburgers, you'll: Take direction from the lead chef in the preparation of orders; Be responsible for prepping ingredients and assembling dishes according to restaurant recipes and specific ...
-
Senior Designer
Found in: Jooble US O C2 - 2 days ago
Turner Duckworth San Francisco, CA, United StatesDesign is central to everything we do at Turner Duckworth, and we're looking for a Senior Designer to join our San Francisco Studio. · In this role, you'll support the Design Directors and your fellow Designers to help build and shape brands to become utterly distinctive and dee ...
-
Caregiver
Found in: One Red Cent US C2 - 3 days ago
Sunrise Senior Living North Atlanta, United StatesSunrise Senior Living was again certified as a Great Place to Work by Activated Insights. This is the 6th time Sunrise has received this top culture and workplace designation, highlighting the special place Sunrise is to be a part of. · COMMUNITY NAME · Sunrise at Buckhead · Job ...
Comments